Hi People!
This is my first blog i am writing here. I will try my best to help you people in understanding and learning #ReactNative.
React Native:
React Native lets you build mobile apps using only #JavaScript. It uses the same design as #React, letting you compose a rich mobile UI using declarative components. So basically react native framework is adding wrappers over native components of IOS and android. So person can write apps for IOS/Android using simple Java Script. I this is much to understand the react native purpose.
One more thing react native current stable version is 0.57
To install Node on MAC:
brew install node
And to install #Homebrew:
To install Homebrew just open Terminal and type ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)". You’ll see messages in the Terminal explaining what you need to do to complete the installation process.
Assuming that you have #Node installed, you can use #npm to install the #Expo CLI command line utility:
npm install -g expo-cli
Then run the following commands to create a new React Native project called "AkaroProject":
expo init AkaroProject
cd AkaroProject
npm start
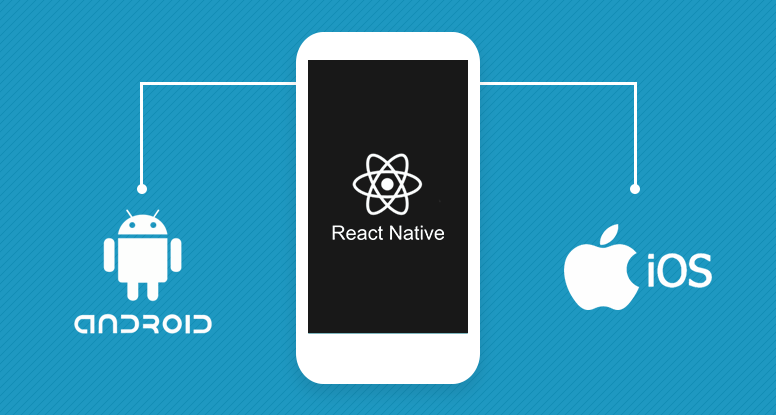
Now your react development server is started for you.
Basics
React Native is like #React, but it uses native components instead of web components as building blocks. So to understand the basic structure of a React Native app, you need to understand some of the basic React concepts, like #JSX, components, state, and props. If you already know React, you still need to learn some React-Native-specific stuff, like the native components. This tutorial is aimed at all audiences, whether you have React experience or not.
Let's do this thing.
Now let's do some magic work:
Add the below code in App.js
import React, { Component } from 'react';
import { Text, View } from 'react-native';
export default class AkaroApp extends Component {
render() {
return (
<View>
<Text>Hello Akaro!</Text>
</View>
);
}
}
import, from, class, and extends in the example above are all ES2015 features. ES2015 (also known as ES6) is a set of improvements to JavaScript that is now part of the official standard.
Code <View><Text>Hello world!</Text></View> is JSX - a syntax for embedding XML within JavaScript. Many frameworks use a special templating language which lets you embed code inside markup language.
In React, this is reversed. JSX lets you write your markup language inside code. It looks like HTML on the web, except instead of web things like <div> or <span>, you use React components. In this case, <Text> is a built-in component that just displays some text and View is like the <div> or <span>.
You'll be making new components a lot. Anything you see on the screen is some sort of component. A component can be pretty simple - the only thing that's required is a render function which returns some JSX to render.
Lets Get Familiar With Building Blocks of React Native App Dev
Props:
Most components can be customised when they are created, with different parameters. These creation parameters are called props. In simple words these are attributes of #reactnative controls. For example UITextField has .text, .placeHolder, .textColour etc.
One basic React Native component is the Image. When you create an image, you can use a prop named source to control what image it shows
import React, { Component } from 'react';
import { AppRegistry, Image } from 'react-native';
export default class Bananas extends Component {
render() {
let pic = {
uri: 'https://www.gstatic.com/webp/gallery3/2.png'
};
return (
<Image source={pic} style={{width: 193, height: 110}}/>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => Bananas);
Braces surrounding {pic} - these embed the variable pic into JSX. You can put any JavaScript expression inside braces in JSX.
Own Custom Components:
Your own components can also use props. This lets you make a single component that is used in many different places in your app, with slightly different properties in each place. Just refer to this.props in your render function.
Here's an example:
import React, { Component } from 'react';
import { AppRegistry, Text, View } from 'react-native';
class Greeting extends Component {
render() {
return (
<View style={{alignItems: 'center'}}>
<Text>Hello {this.props.name}!</Text>
</View>
);
}
}
export default class LotsOfGreetings extends Component {
render() {
return (
<View style={{alignItems: 'center'}}>
<Greeting name='Rakesh' />
<Greeting name='Ajay' />
<Greeting name='Shiva' />
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => LotsOfGreetings);
Using name as a prop lets us customise the Greeting component, so we can reuse that component for each of our greetings.
State:
There are two types of data that control a component: props and state. props are set by the parent and they are fixed throughout the lifetime of a component. For data that is going to change, we have to use state.
In general, you should initialise state in the constructor, and then call setState when you want to change it. So in simple term props equivalent to let and State equivalent to var of swift we can say.
Example:
For example, let's say we want to make text that blinks all the time. The text itself gets set once when the blinking component gets created, so the text itself is a prop. The "whether the text is currently on or off" changes over time, so that should be kept in state.
import React, { Component } from 'react';
import { AppRegistry, Text, View } from 'react-native';
class Blink extends Component {
constructor(props) {
super(props);
this.state = { isShowingText: true };
// Toggle the state every second
setInterval(() => (
this.setState(previousState => (
{ isShowingText: !previousState.isShowingText }
))
), 1000);
}
render() {
if (!this.state.isShowingText) {
return null;
}
return (
<Text>{this.props.text}</Text>
);
}
}
export default class BlinkApp extends Component {
render() {
return (
<View>
<Blink text='I love to blink' />
<Blink text='Yes blinking is so great' />
<Blink text='Why did they ever take this out of HTML' />
<Blink text='Look at me look at me look at me' />
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => BlinkApp);
In a real application, you probably won't be setting state with a timer. You might set state when you have new data arrived from the server, or from user input
Style:
You just #style your application using JavaScript. All of the core components accept a prop named style. The style names and values usually match how #CSS works on the web, except names are written using camel casing, e.g #backgroundColor rather than background-color.
As a component grows in complexity, it is often cleaner to use StyleSheet.create to define several styles in one place. Here's an example:
import React, { Component } from 'react';
import { AppRegistry, StyleSheet, Text, View } from 'react-native';
const styles = StyleSheet.create({
bigblue: {
color: 'blue',
fontWeight: 'bold',
fontSize: 30,
},
red: {
color: 'red',
},
});
export default class LotsOfStyles extends Component {
render() {
return (
<View>
<Text style={styles.red}>just red</Text>
<Text style={styles.bigblue}>just bigblue</Text>
<Text style={[styles.bigblue, styles.red]}>bigblue, then red</Text>
<Text style={[styles.red, styles.bigblue]}>red, then bigblue</Text>
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => LotsOfStyles);
Fixed Dimensions:
The simplest way to set the dimensions of a component is by adding a fixed width and height to style. All dimensions in #ReactNative are unitless, and represent #density-independent #pixels.
import React, { Component } from 'react';
import { AppRegistry, View } from 'react-native';
export default class FixedDimensionsBasics extends Component {
render() {
return (
<View>
<View style={{width: 50, height: 50, backgroundColor: 'powderblue'}} />
<View style={{width: 100, height: 100, backgroundColor: 'skyblue'}} />
<View style={{width: 150, height: 150, backgroundColor: 'steelblue'}} />
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => FixedDimensionsBasics);
Flex Dimensions:
Use flex in a component's style to have the component expand and shrink dynamically based on available space. Normally you will use flex: 1, which tells a component to fill all available space, shared evenly amongst other components with the same parent. The larger the flex given, the higher the ratio of space a component will take compared to its siblings.
Note: A component can only expand to fill available space if its parent has dimensions greater than 0. If a parent does not have either a fixed width and height or flex, the parent will have dimensions of 0 and the flex children will not be visible.
import React, { Component } from 'react';
import { AppRegistry, View } from 'react-native';
export default class FlexDimensionsBasics extends Component {
render() {
return (
// Try removing the `flex: 1` on the parent View.
// The parent will not have dimensions, so the children can't expand.
// What if you add `height: 300` instead of `flex: 1`?
<View style={{flex: 1}}>
<View style={{flex: 1, backgroundColor: 'powderblue'}} />
<View style={{flex: 2, backgroundColor: 'skyblue'}} />
<View style={{flex: 3, backgroundColor: 'steelblue'}} />
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => FlexDimensionsBasics);
Layout with Flexbox:
A component can specify the layout of its children using the flexbox algorithm. Flexbox is designed to provide a consistent layout on different screen sizes.
Note: The defaults are with flexDirection defaulting to column , and the flexparameter only supporting a single number.
Flex Direction:
Adding flexDirection to a component's style determines the primary axis of its layout. Should the children be organized horizontally (row) or vertically (column)? The default is column.
import React, { Component } from 'react';
import { AppRegistry, View } from 'react-native';
export default class FlexDirectionBasics extends Component {
render() {
return (
// Try setting `flexDirection` to `column`.
<View style={{flex: 1, flexDirection: 'row'}}>
<View style={{width: 50, height: 50, backgroundColor: 'powderblue'}} />
<View style={{width: 50, height: 50, backgroundColor: 'skyblue'}} />
<View style={{width: 50, height: 50, backgroundColor: 'steelblue'}} />
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => FlexDirectionBasics);
Justify Content:
Adding justifyContent to a component's style determines the distribution of children along the primary axis. Should children be distributed at the start, the center, the end, or spaced evenly? Available options are flex-start, center, flex-end, space-around, space-between and space-evenly.
import React, { Component } from 'react';
import { AppRegistry, View } from 'react-native';
export default class JustifyContentBasics extends Component {
render() {
return (
// Try setting `justifyContent` to `center`.
// Try setting `flexDirection` to `row`.
<View style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'space-between',
}}>
<View style={{width: 50, height: 50, backgroundColor: 'powderblue'}} />
<View style={{width: 50, height: 50, backgroundColor: 'skyblue'}} />
<View style={{width: 50, height: 50, backgroundColor: 'steelblue'}} />
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => JustifyContentBasics);
Align Items:
Adding alignItems to a component's style determines the alignment of children along the secondary axis (if the primary axis is row, then the secondary is column, and vice versa). Should children be aligned at the start, the center, the end, or stretched to fill? Available options are flex-start, center, flex-end, and stretch.
Note:For stretch to have an effect, children must not have a fixed dimension along the secondary axis. In the following example, setting alignItems: stretch does nothing until the width: 50 is removed from the children.
import React, { Component } from 'react';
import { AppRegistry, View } from 'react-native';
export default class AlignItemsBasics extends Component {
render() {
return (
// Try setting `alignItems` to 'flex-start'
// Try setting `justifyContent` to `flex-end`.
// Try setting `flexDirection` to `row`.
<View style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'center',
alignItems: 'stretch',
}}>
<View style={{width: 50, height: 50, backgroundColor: 'powderblue'}} />
<View style={{height: 50, backgroundColor: 'skyblue'}} />
<View style={{height: 100, backgroundColor: 'steelblue'}} />
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => AlignItemsBasics);
Handling TextField (TextInput):
#TextInput is a basic component that allows the user to enter text. It has an onChangeText prop that takes a function to be called every time the text changed, and an onSubmitEditing prop that takes a function to be called when the text is submitted.
Lets take simple example as below:
import React, { Component } from 'react';
import { AppRegistry, Text, TextInput, View } from 'react-native';
export default class PizzaTranslator extends Component {
constructor(props) {
super(props);
this.state = {text: ''};
}
render() {
return (
<View style={{padding: 10}}>
<TextInput
style={{height: 40}}
placeholder="Type here to translate!"
onChangeText={(text) => this.setState({text})}
/>
<Text style={{padding: 10, fontSize: 42}}>
{this.state.text.split(' ').map((word) => word && 'Akaro').join(' ')}
</Text>
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => PizzaTranslator);
Above example translates the typed text word into "Akaro" word.
Handling Touches
Users interact with #mobile apps mainly through touch. They can use a combination of #gestures, such as #tapping on a button, #scrolling a list, or #zooming on a #map. #React #Native provides components to handle all sorts of common gestures, as well as a comprehensive gesture responder system to allow for more advanced gesture recognition, but the one component you will most likely be interested in is the basic Button.
Displaying a basic button
Button provides a basic button component that is rendered nicely on all platforms. The minimal example to display a button looks like this:
<Button
onPress={() => {
Alert.alert('You tapped the button!');
}}
title="Press Me"
/>
This will render a blue label on iOS, and a blue rounded rectangle with white text on Android. Pressing the button will call the "onPress" function, which in this case displays an alert popup. If you like, you can specify a "color" prop to change the color of your button.
import React, { Component } from 'react';
import { Alert, AppRegistry, Button, StyleSheet, View } from 'react-native';
export default class ButtonBasics extends Component {
_onPressButton() {
Alert.alert('You tapped the button!')
}
render() {
return (
<View style={styles.container}>
<View style={styles.buttonContainer}>
<Button
onPress={this._onPressButton}
title="Press Me"
/>
</View>
<View style={styles.buttonContainer}>
<Button
onPress={this._onPressButton}
title="Press Me"
color="#841584"
/>
</View>
<View style={styles.alternativeLayoutButtonContainer}>
<Button
onPress={this._onPressButton}
title="This looks great!"
/>
<Button
onPress={this._onPressButton}
title="OK!"
color="#841584"
/>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
},
buttonContainer: {
margin: 20
},
alternativeLayoutButtonContainer: {
margin: 20,
flexDirection: 'row',
justifyContent: 'space-between'
}
});
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => ButtonBasics);
Above example declare one method named _onPressButton and which called by button press event of each button of class
Touchables
If the basic button doesn't look right for your app, you can build your own button using any of the #Touchable components provided by React Native. The #Touchable components provide the capability to capture tapping gestures, and can display feedback when a #gesture is recognized. These components do not provide any default styling, however, so you will need to do a bit of work to get them looking nicely in your app.
Touchables Categories:
Which "Touchable" component you use will depend on what kind of feedback you want to provide:
Generally, you can use #TouchableHighlight anywhere you would use a button or link on web. The view's background will be darkened when the user presses down on the button.
You may consider using #TouchableNativeFeedback on Android to display ink surface reaction ripples that respond to the user's touch.
#TouchableOpacity can be used to provide feedback by reducing the opacity of the button, allowing the background to be seen through while the user is pressing down.
If you need to handle a tap gesture but you don't want any feedback to be displayed, use #TouchableWithoutFeedback.
In some cases, you may want to detect when a user presses and holds a view for a set amount of time. These long presses can be handled by passing a function to the onLongPress props of any of the "Touchable" components.
See below example:
import React, { Component } from 'react';
import { Alert, AppRegistry, Platform, StyleSheet, Text, TouchableHighlight, TouchableOpacity, TouchableNativeFeedback, TouchableWithoutFeedback, View } from 'react-native';
export default class Touchables extends Component {
_onPressButton() {
Alert.alert('You tapped the button!')
}
_onLongPressButton() {
Alert.alert('You long-pressed the button!')
}
render() {
return (
<View style={styles.container}>
<TouchableHighlight onPress={this._onPressButton} underlayColor="white">
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableHighlight</Text>
</View>
</TouchableHighlight>
<TouchableOpacity onPress={this._onPressButton}>
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableOpacity</Text>
</View>
</TouchableOpacity>
<TouchableNativeFeedback
onPress={this._onPressButton}
background={Platform.OS === 'android' ? TouchableNativeFeedback.SelectableBackground() : ''}>
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableNativeFeedback</Text>
</View>
</TouchableNativeFeedback>
<TouchableWithoutFeedback
onPress={this._onPressButton}
>
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableWithoutFeedback</Text>
</View>
</TouchableWithoutFeedback>
<TouchableHighlight onPress={this._onPressButton} onLongPress={this._onLongPressButton} underlayColor="white">
<View style={styles.button}>
<Text style={styles.buttonText}>Touchable with Long Press</Text>
</View>
</TouchableHighlight>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
paddingTop: 60,
alignItems: 'center'
},
button: {
marginBottom: 30,
width: 260,
alignItems: 'center',
backgroundColor: '#2196F3'
},
buttonText: {
padding: 20,
color: 'white'
}
});
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => Touchables);
Using a ScrollView
The #ScrollView is a generic #scrolling #container that can host multiple components and views. The #scrollable items need not be #homogeneous, and you can scroll both vertically and horizontally (by setting the #horizontal property).
Below example creates a #vertical #ScrollView with both images and text mixed together.
export default class IScrolledDownAndWhatHappenedNextShockedMe extends Component {
render() {
return (
<ScrollView>
<Text style={{fontSize:96}}>Scroll me plz</Text>
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Text style={{fontSize:96}}>If you like</Text>
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Text style={{fontSize:96}}>Scrolling down</Text>
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Text style={{fontSize:96}}>What's the best</Text>
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Text style={{fontSize:96}}>Framework around?</Text>
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Image source={{uri: "https://facebook.github.io/react-native/img/favicon.png", width: 64, height: 64}} />
<Text style={{fontSize:80}}>React Native</Text>
</ScrollView>
);
}
}
// skip these lines if using Create React Native App
AppRegistry.registerComponent(
'AkaroProject',
() => IScrolledDownAndWhatHappenedNextShockedMe);
Note:ScrollViews can be configured to allow paging through views using swiping gestures by using the pagingEnabled props. Swiping horizontally between views can also be implemented on Android using the ViewPagerAndroid component.
Note:A ScrollView with a single item can be used to allow the user to zoom content. Set up the maximumZoomScale and minimumZoomScale props and your user will be able to use pinch and expand gestures to zoom in and out.
Using List Views
React Native provides a suite of components for presenting lists of data. Generally, you'll want to use either #FlatList or #SectionList.
The FlatList component displays a scrolling list of changing, but similarly structured, data. #FlatListworks well for long lists of data, where the number of items might change over time. Unlike the more generic #ScrollView, the #FlatList only renders elements that are currently showing on the screen, not all the elements at once.
The #FlatList component requires two props: #data and #renderItem. data is the source of information for the list. renderItem takes one item from the source and returns a formatted component to render.
This example creates a simple #FlatList of hardcoded data. Each item in the data props is rendered as a Text component. The #FlatListBasics component then renders the FlatList and all #Textcomponents.
Here you go for simple Flat list (Plain TableView in IOS) example:
import React, { Component } from 'react';
import { AppRegistry, FlatList, StyleSheet, Text, View } from 'react-native';
export default class FlatListBasics extends Component {
render() {
return (
<View style={styles.container}>
<FlatList
data={[
{key: 'Devin'},
{key: 'Jackson'},
{key: 'James'},
{key: 'Joel'},
{key: 'John'},
{key: 'Jillian'},
{key: 'Jimmy'},
{key: 'Julie'},
]}
renderItem={({item}) => <Text style={styles.item}>{item.key}</Text>}
/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: 22
},
item: {
padding: 10,
fontSize: 18,
height: 44,
},
})
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => FlatListBasics);
If you want to render a set of data broken into logical sections, maybe with #section #headers, similar to #UITableViews on #iOS, then a #SectionList is the way to go.
import React, { Component } from 'react';
import { AppRegistry, SectionList, StyleSheet, Text, View } from 'react-native';
export default class SectionListBasics extends Component {
render() {
return (
<View style={styles.container}>
<SectionList
sections={[
{title: 'D', data: ['Devin']},
{title: 'J', data: ['Jackson', 'James', 'Jillian', 'Jimmy', 'Joel', 'John', 'Julie']},
]}
renderItem={({item}) => <Text style={styles.item}>{item}</Text>}
renderSectionHeader={({section}) => <Text style={styles.sectionHeader}>{section.title}</Text>}
keyExtractor={(item, index) => index}
/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: 22
},
sectionHeader: {
paddingTop: 2,
paddingLeft: 10,
paddingRight: 10,
paddingBottom: 2,
fontSize: 14,
fontWeight: 'bold',
backgroundColor: 'rgba(247,247,247,1.0)',
},
item: {
padding: 10,
fontSize: 18,
height: 44,
},
})
// skip this line if using Create React Native App
AppRegistry.registerComponent('AkaroProject', () => SectionListBasics);
Comments