Paytm Integration IOS Swift FrameWork
- Rakesh Kumar
- Feb 16, 2019
- 3 min read
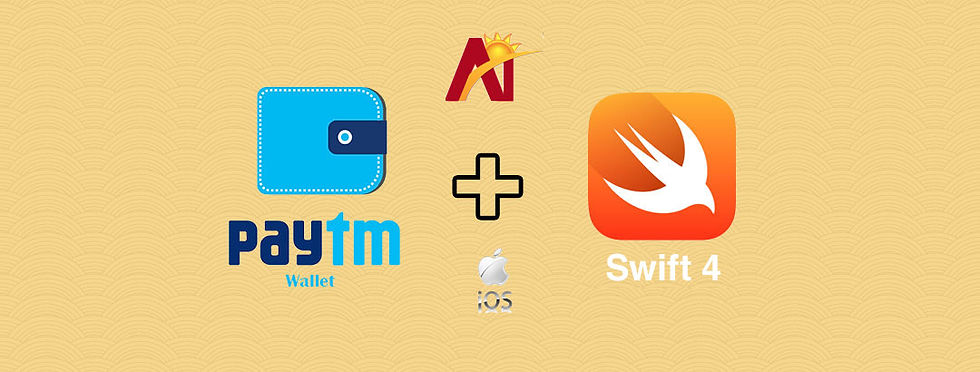
Let's go step by step how i implemented.
First of all you need to create your own paytm merchant account:
Then go to https://dashboard.paytm.com/next/get-started and click on "Setup #Payment #Gateway" in Payment Gateway section:
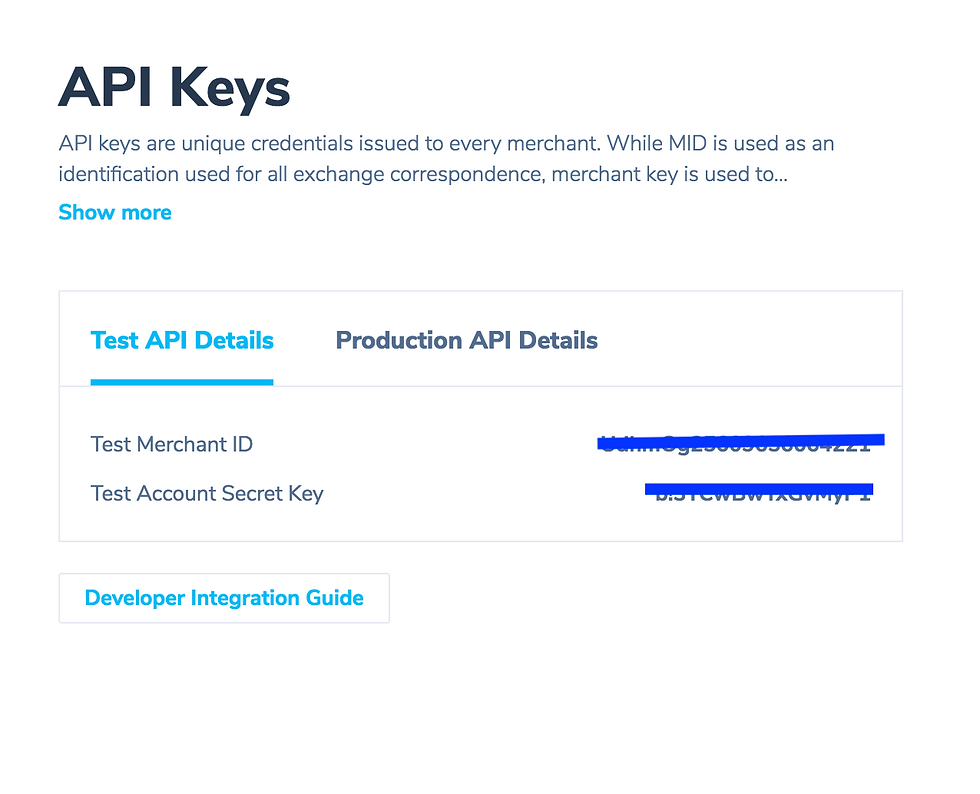
You will get your test merchant key and account secret key. Don't share your MERCHANT_KEY. Its unique and used by paytm to do transactions for your account.
Now let move to code section. You can download the sample code by visiting below link:
https://developer.paytm.com/docs/v1/ios-sdk
I have used swift PaytmSDK.framework so my next steps will be based upon this.
After downloading the sample code from https://github.com/Paytm-Payments/Paytm_iOS_App_Kit
Copy the framework #PaymentSDK.framework into your project and add it to your project by dragging in file explorer bar of Xcode
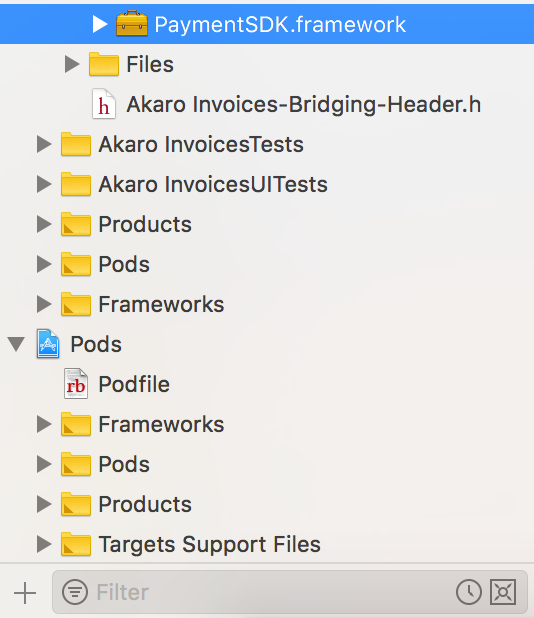
Then in your project target's build phases section add #SystemConfiguration.framework under "Link Binary With Libraries " section and if #PaytmSDK.framework is not there then add it by pressing + button.
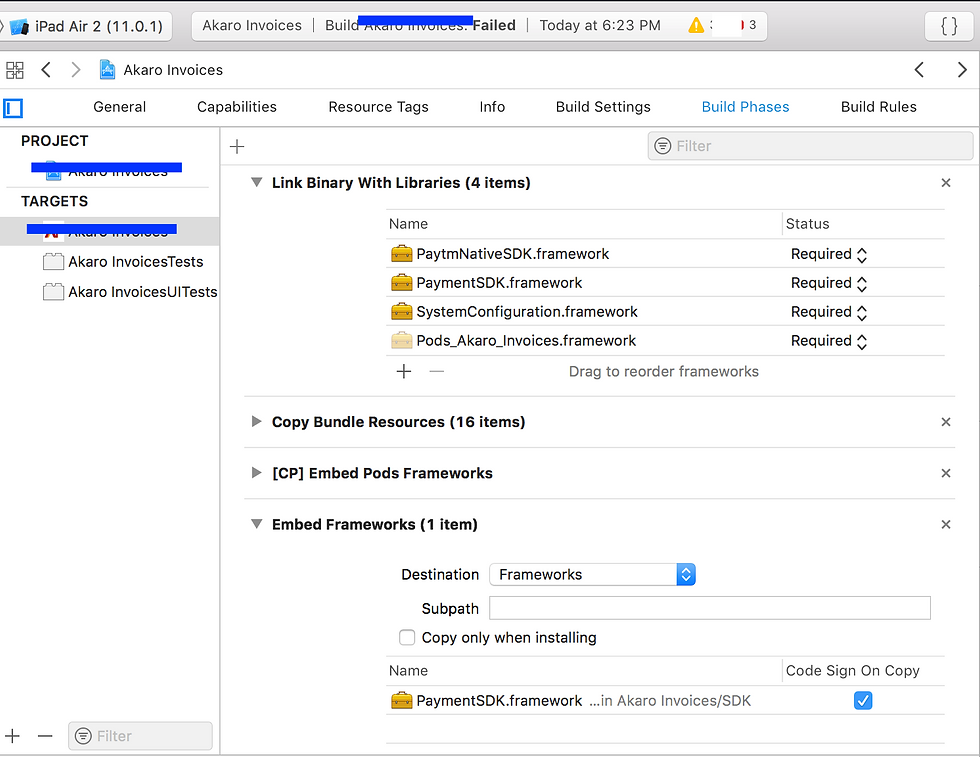
Also add PaytmSDK.framework in build phases section of your project target under "#Embed #Frameworks" section. If "Embed Frameworks" section is not found then add one by hitting + button:
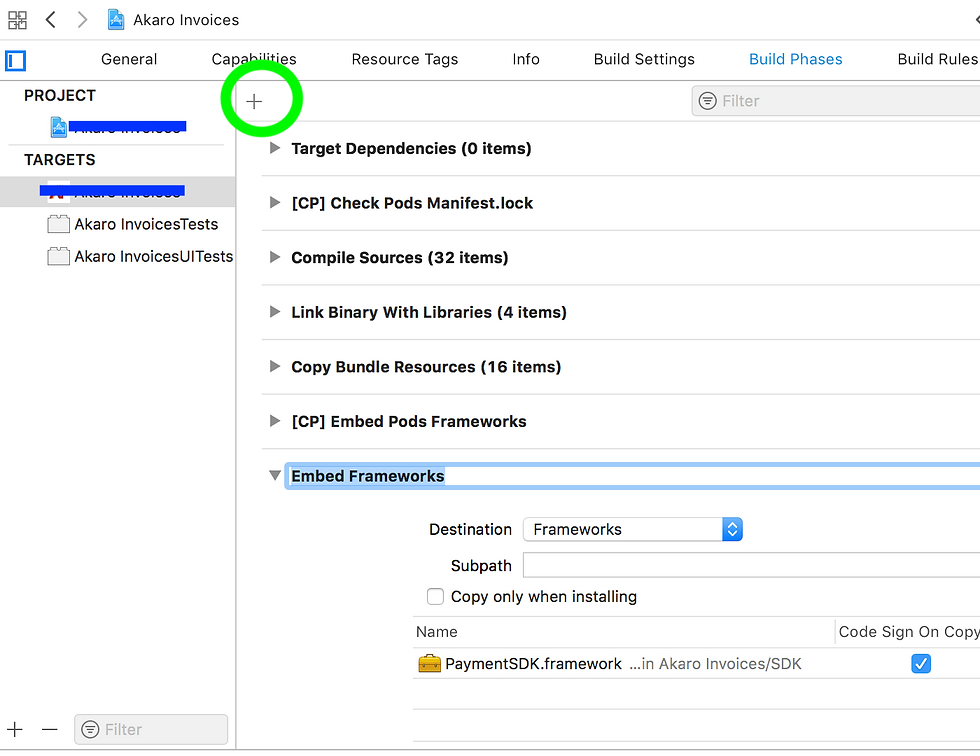
Now framwork is added then import PaymentSDK in your view controller:
import PaymentSDK
Then add below extension to your viewcontroller:
extension AIPaymentSettingsVC :PGTransactionDelegate{
func didFinishedResponse(_ controller: PGTransactionViewController, response responseString: String) {
print(responseString)
}
func didCancelTrasaction(_ controller: PGTransactionViewController) {
print("CANCELLED")
self.removeController(controller: controller)
}
func errorMisssingParameter(_ controller: PGTransactionViewController, error: NSError?) {
self.removeController(controller: controller)
if error != nil {
print(error?.localizedDescription ?? "")
}
}
func showController(controller: PGTransactionViewController) {
if self.navigationController != nil {
self.navigationController?.pushViewController(controller, animated: true)
} else {
self.present(controller, animated: true, completion: nil)
}
}
func removeController(controller: PGTransactionViewController) {
if self.navigationController != nil {
self.navigationController?.popViewController(animated: true)
} else {
controller.dismiss(animated: true, completion: nil)
}
}
@objc func initialisePayment() {
let custId = UUID().uuidString
let orderId = UUID().uuidString
var orderDict = [String : Any]()
orderDict["MID"] = "Your merchant Id"
orderDict["ORDER_ID"] = orderId
orderDict["CUST_ID"] = custId
orderDict["MOBILE_NO"] = "Your Mobile Number"
orderDict["EMAIL"] = "Your Email Id"
orderDict["INDUSTRY_TYPE_ID"] = "Retail";
orderDict["CHANNEL_ID"] = "WAP";
orderDict["TXN_AMOUNT"] = "1.00"
orderDict["WEBSITE"] = "WEBSTAGING";
orderDict["CALLBACK_URL"] = "
https://securegw-stage.paytm.in/theia/paytmCallback?ORDER_ID="+ orderId
orderDict["CHECKSUMHASH"] = "";
API.shared.generateChecksumHashCall(userInfo: orderDict) { (response, error) in
SVProgressHUD.dismiss()
if error == nil {
self.creatPayment(order: response as! Dictionary<String, Any>)
}
}
}
//Creat Payment----------------
func creatPayment(order:Dictionary<String, Any>) {
let mc = PGMerchantConfiguration.defaultMerchantObject
let transaction = PGTransactionViewController.init(transactionParameters: order)
transaction.serverType = PaymentSDK.ServerType.eServerTypeStaging
transaction.merchant = mc
transaction.delegate = self
self.navigationController?.pushViewController(transaction, animated: true)
}
}
So all done on IOS Side. Now You have to add server side code to generate and verify Checksumhash
in any language of your choice. I have done in #Node-JS
Download the #node -JS source code from below URL:
https://github.com/Paytm-Payments/Paytm_App_Checksum_Kit_NodeJs

Add your router for generating/verifying paytm #checksum:
var express = require('express');
var router = express.Router();
var paytm_config = require('../paytm/paytm_config').paytm_config;
var paytm_checksum = require('../paytm/checksum');
var querystring = require('querystring');
router.post('/api', function(request, response) {
console.log("/ has started");
response.writeHead(200 , {'Content-type':'text/html'});
response.write('<html><head><title>Paytmdddddd</title></head><body>');
response.write('</body></html>');
response.end();
})
router.post('/api/generate_checksum', function(request, response) {
var paramarray = {};
paramarray['MID'] = request.body.MID; //Provided by Paytm
paramarray['ORDER_ID'] = request.body.ORDER_ID; //unique OrderId for every request
paramarray['CUST_ID'] = request.body.CUST_ID; // unique customer identifier
paramarray['INDUSTRY_TYPE_ID'] = request.body.INDUSTRY_TYPE_ID; //Provided by Paytm
paramarray['CHANNEL_ID'] = request.body.CHANNEL_ID; //Provided by Paytm
paramarray['TXN_AMOUNT'] = request.body.TXN_AMOUNT; // transaction amount
paramarray['WEBSITE'] = request.body.WEBSITE; //Provided by Paytm
paramarray['CALLBACK_URL'] = request.body.CALLBACK_URL;//Provided by Paytm
paramarray['EMAIL'] = request.body.EMAIL; // customer email id
paramarray['MOBILE_NO'] = request.body.MOBILE_NO; // customer 10 digit mobile no.
paytm_checksum.genchecksum(paramarray, paytm_config.MERCHANT_KEY, function (err, res) {
response.writeHead(200, {'Content-type' : 'text/json','Cache-Control': 'no-cache'});
response.write(JSON.stringify(res));
response.end();
});
})
router.post('/api/verify_checksum', function(request, response) {
if(request.method == 'POST'){
var fullBody = '';
request.on('data', function(chunk) {
fullBody += chunk.toString();
});
request.on('end', function() {
var decodedBody = querystring.parse(fullBody);
response.writeHead(200, {'Content-type' : 'text/html','Cache-Control': 'no-cache'});
if(paytm_checksum.verifychecksum(decodedBody, paytm_config.MERCHANT_KEY)) {
console.log("true");
}else{
console.log("false");
}
// if checksum is validated Kindly verify the amount and status
// if transaction is successful
// kindly call Paytm Transaction Status API and verify the transaction amount and status.
// If everything is fine then mark that transaction as successful into your DB.
response.end();
});
}else{
response.writeHead(200, {'Content-type' : 'text/json'});
response.end();
}
})
module.exports = router;
Note: Make sure you have changed the keys with your paytm developer account key in "paytm_config.js"
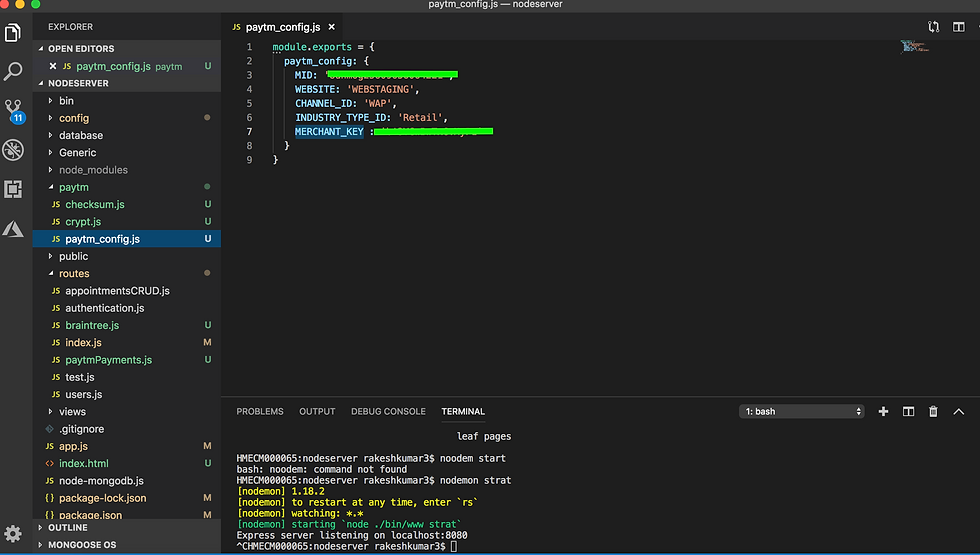
After generating the checksum-hash from node server call will go to paytm server and you will see paytm payment screen on your app screen.
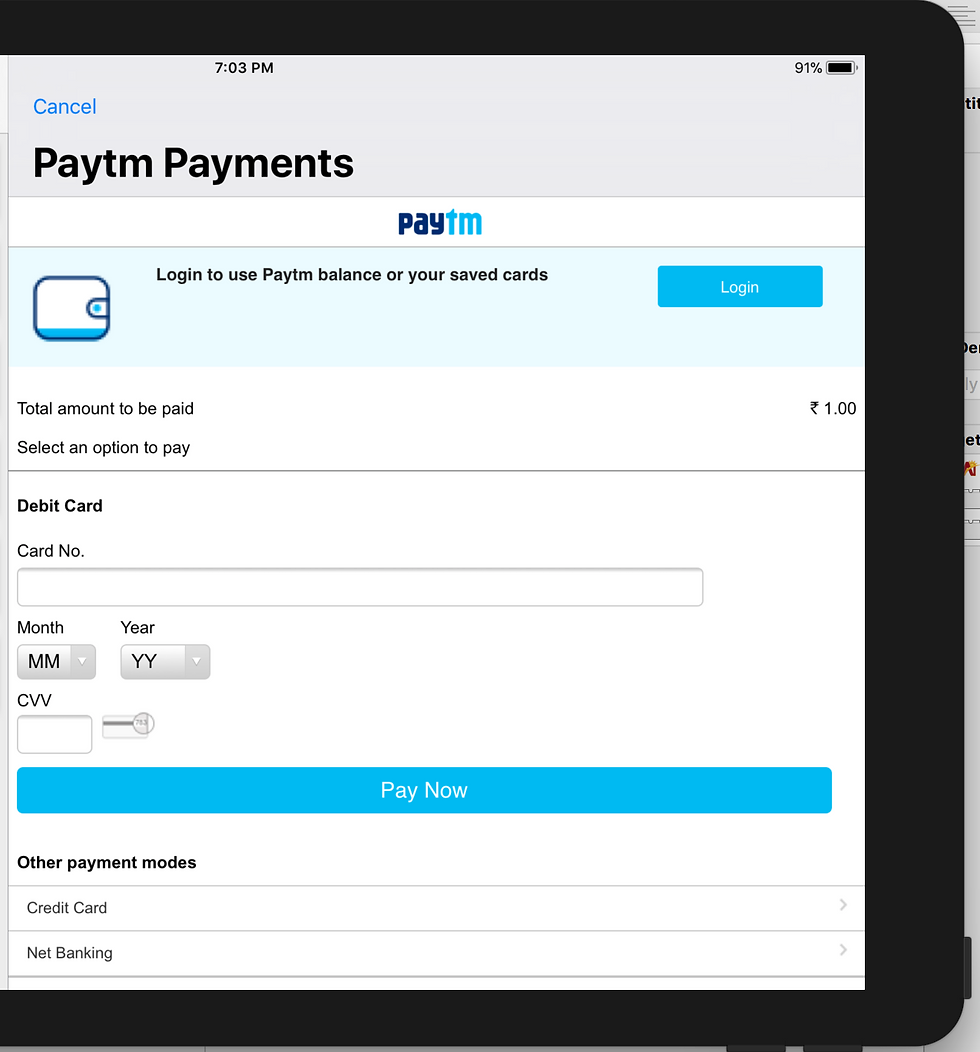
Happy coding...
#NodeJS #IOS #Swift #Paytm #Payment #PaymentSDK.framework #Framework
Comments