React Native Networking
- Rakesh Kumar
- Jan 30, 2019
- 3 min read
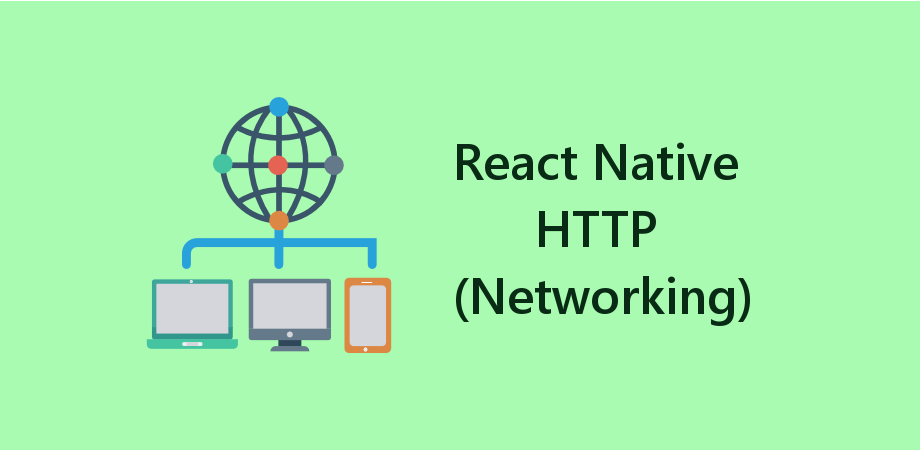
How to send and receive data to and from server is the brief of this article. Its an interesting and important topic so i am covering this separately. As i have provided the details about react native installation, building projects and basic components in other blog https://www.akarotech.com/blog/categories/react-native
Lets start the concept of networking with React Native without any delay :)
Networking
Many mobile apps need to load resources from a #remote #URL. You may want to make a #POST request to a #REST #API, or you may simply need to fetch a chunk of static content from another server.
Using Fetch
#React #Native provides the #Fetch #API for your networking needs. Fetch will seem familiar if you have used #XMLHttpRequest or other networking #APIs before. You may refer to #MDN's guide on #Using #Fetch for additional information.
Making requests
fetch('https://mywebsite.com/mydata.json');
#Fetch also takes an optional second argument that allows you to customize the #HTTP request. You may want to specify additional headers, or make a #POST request:
fetch('https://mywebsite.com/endpoint/', {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
firstParam: 'yourValue1',
secondParam: 'yourValue2',
}),
});
Take a look at the Fetch Request docs for a full list of properties.
Handling the response
The above examples show how you can make a request. In many cases, you will want to do something with the response.
#Networking is an inherently #asynchronous #operation. #Fetch methods will return a #Promise that makes it straightforward to write code that works in an #asynchronous manner:
function getMoviesFromApiAsync() {
return fetch('https://facebook.github.io/react-native/movies.json')
.then((response) => response.json())
.then((responseJson) => {
return responseJson.movies;
})
.catch((error) => {
console.error(error);
});
}
You can also use the proposed #ES2017 async/await syntax in a React Native app:
async function getMoviesFromApi() {
try {
let response = await fetch(
'https://facebook.github.io/react-native/movies.json',
);
let responseJson = await response.json();
return responseJson.movies;
} catch (error) {
console.error(error);
}
}
Don't forget to catch any errors that may be thrown by fetch, otherwise they will be dropped silently.
import React from 'react';
import { FlatList, ActivityIndicator, Text, View } from 'react-native';
export default class FetchExample extends React.Component {
constructor(props){
super(props);
this.state ={ isLoading: true}
}
componentDidMount(){
return fetch('https://facebook.github.io/react-native/movies.json')
.then((response) => response.json())
.then((responseJson) => {
this.setState({
isLoading: false,
dataSource: responseJson.movies,
}, function(){
});
})
.catch((error) =>{
console.error(error);
});
}
render(){
if(this.state.isLoading){
return(
<View style={{flex: 1, padding: 20}}>
<ActivityIndicator/>
</View>
)
}
return(
<View style={{flex: 1, paddingTop:20}}>
<FlatList
data={this.state.dataSource}
renderItem={({item}) => <Text>{item.title}, {item.releaseYear}</Text>}
keyExtractor={({id}, index) => id}
/>
</View>
);
}
}
Using Other Networking Libraries
The #XMLHttpRequest API is built in to React Native. This means that you can use third party libraries such as #frisbee or #axios that depend on it, or you can use the #XMLHttpRequest API directly if you prefer.
var request = new XMLHttpRequest();
request.onreadystatechange = (e) => {
if (request.readyState !== 4) {
return;
}
if (request.status === 200) {
console.log('success', request.responseText);
} else {
console.warn('error');
}
};
request.open('GET', 'https://mywebsite.com/endpoint/');
request.send();
The security model for #XMLHttpRequest is different than on web as there is no concept of #CORS in native apps.
WebSocket Support
React Native also supports #WebSockets, a protocol which provides full-duplex communication channels over a single #TCP connection.
var ws = new WebSocket('ws://host.com/path');
ws.onopen = () => {
// connection opened
ws.send('something'); // send a message
};
ws.onmessage = (e) => {
// a message was received
console.log(e.data);
};
ws.onerror = (e) => {
// an error occurred
console.log(e.message);
};
ws.onclose = (e) => {
// connection closed
console.log(e.code, e.reason);
};
Congrats. Next, you might want to check out all the cool stuff the community does with React Native. https://facebook.github.io/react-native/docs/components-and-apis
Source of Data:
https://facebook.github.io/react-native/docs/getting-started
Note: I have simplified the contents as notes, so that beginners can understand it easily. I will try to upload video soon...
Comments