Large Size Title Swift IOS 11
- Rakesh Kumar
- Feb 15, 2019
- 1 min read
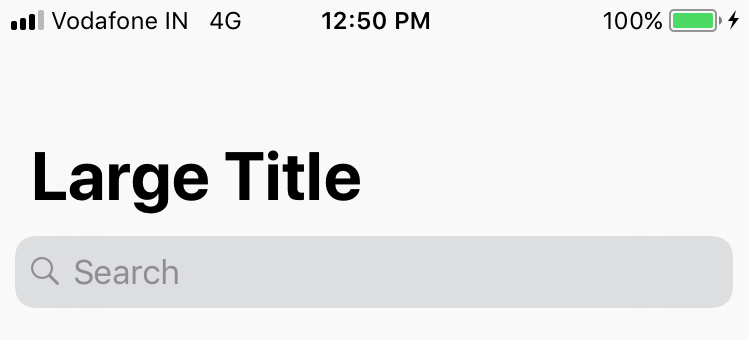
Declare the search controller as property of Your View Controller:
let searchController = UISearchController(searchResultsController: nil)
var inventoryList = Array<EntityInventory>()
var inventoryListSearched = Array<EntityInventory>()
Then Call below method from viewDidLoad:
func setup() {
if #available(iOS 11.0, *) {
self.navigationController?.navigationBar.prefersLargeTitles = true
self.navigationItem.largeTitleDisplayMode = .automatic
searchController.delegate = self
searchController.searchResultsUpdater = self
searchController.dimsBackgroundDuringPresentation = false
self.navigationItem.searchController = searchController
} else {
// Fallback on earlier versions
}
}
Then define the tableview delegates as below:
// MARK: - Table View
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.inventoryListSearched.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "inventoryCell", for: indexPath)
let inventoryObj = self.inventoryListSearched[indexPath.row]
configureCell(cell, withItem: inventoryObj, atIndePath:indexPath)
let bgView:UIView = cell.viewWithTag(100) ?? UIView()
bgView.clipsToBounds = false
bgView.layer.shadowColor = UIColor.black.cgColor
bgView.layer.shadowOpacity = 0.3
bgView.layer.shadowOffset = CGSize.zero
bgView.layer.shadowRadius = 1
return cell
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
self.searchController.dismiss(animated: false) {
}
}
func configureCell(_ cell: UITableViewCell, withItem item: EntityInventory, atIndePath:IndexPath) {
let lblName:UILabel = cell.viewWithTag(1) as! UILabel
lblName.text = item.title
let lblEmail:UILabel = cell.viewWithTag(2) as! UILabel
lblEmail.text = item.currency! + "\(item.unitPrice)"
let lblCityState:UILabel = cell.viewWithTag(3) as! UILabel
lblCityState.text = item.inventoryDecripton
}
Then implement search controller delegates
extension YourViewControler:UISearchControllerDelegate, UISearchResultsUpdating {
func updateSearchResults(for searchController: UISearchController) {
var filteredArray = Array<EntityInventory>()
if let searchText = searchController.searchBar.text, !searchText.isEmpty {
filteredArray = self.inventoryList.filter { ($0.title?.lowercased().contains(searchText.lowercased()))! || ($0.inventoryDecripton?.lowercased().contains(searchText.lowercased()))!}
}
else {
filteredArray = self.inventoryList
}
self.inventoryListSearched = filteredArray
tableView.reloadData()
}
There is nothing much to do so not uploading screen shots. Happy coding...
Comments